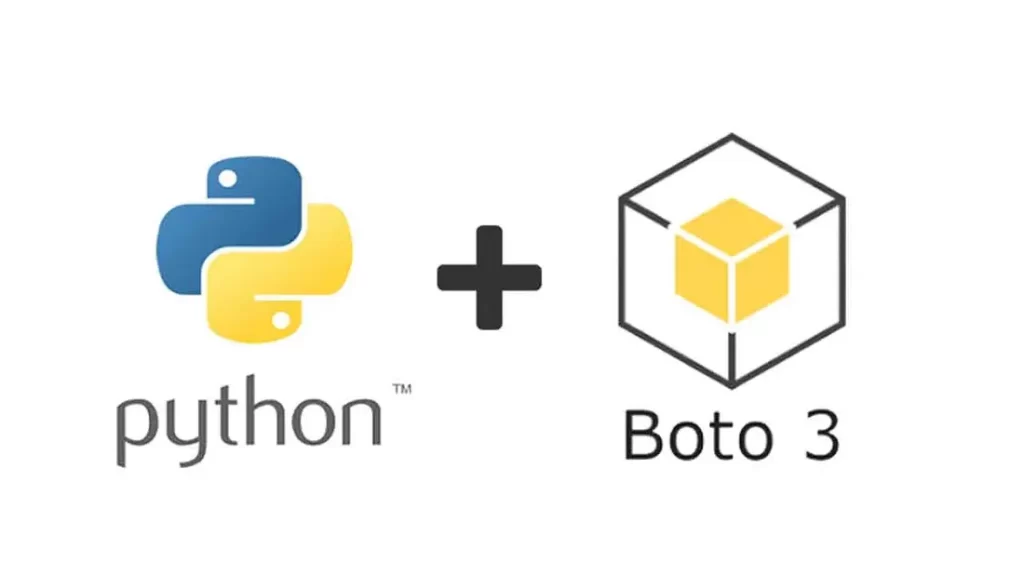
Boto3 is a software development kit (SDK) that allows developers to interact with Amazon Web Services (AWS) services using Python. It is designed to be user-friendly and flexible, making it easy for developers to build, deploy and manage AWS services, including EC2 instances, RDS databases, and S3 storage.
Boto3 includes built-in support for Amazon’s cloud management tools such as Amazon CloudWatch, Amazon S3 Transfer Acceleration, and Amazon SNS. This makes it simple for developers to integrate these tools into their applications without writing extra code.
What sets boto3 apart from other AWS SDKs is its speed and efficiency. AWS has invested in optimizing the performance of boto3 so that developers can interact with AWS services in real-time, without waiting for responses.
Installation
- The prerequisites for installing boto3 are:
- Python version 2.7, 3.4, 3.5, 3.6, or 3.7
- pip package management tool
- AWS account and access key ID and secret access key
- AWS CLI
Once you have met these requirements, to install Boto3, the AWS SDK for Python, you can use pip, the Python package manager. Open your terminal or command prompt and run the following command:
pip install boto3
This will download and install the latest version of Boto3 and its dependencies. Once the installation is complete, you can start using Boto3 in your Python code by importing the library like this:
import boto3
Configuration
To configure Boto 3 using the AWS CLI, you must first ensure that you have the AWS CLI installed as mentioned in the prerequisites and an AWS account. You will then need to create authentication credentials for your AWS account, which can be done through the IAM (Identity and Access Management) Console.
- To access the IAM Console, log in to your AWS account and navigate the IAM dashboard. From there, you can either create a new user or use an existing one. Once you have a user selected, navigate to the “Security Credentials” tab, where you will find the option to “Create access key.”
- Click this option to generate a new set of access keys, which will consist of an Access Key ID and a Secret Access Key. These keys are used to secure access to your AWS resources and should be kept confidential.
- To configure your credentials file using the AWS CLI, open a terminal window and enter the following command:
aws configure
- You will be prompted to enter your AWS Access Key ID, Secret Access Key, and default region name. After entering this information, your credentials file will be created and stored in the default location for your operating system. with the credentials file properly configured, you can now use the AWS CLI to manage your AWS resources and access them through Boto 3.
Alternatively, you can create the credential file yourself. By default, its location is at ~/.aws/credentials:
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
You may also want to set a default region. This can be done in the configuration file. By default, its location is at ~/.aws/config:
[default]
region=us-east-1
Alternatively, you can pass a region_name when creating clients and resources.
This sets up credentials for the default profile as well as a default region to use when creating connections.
Client Versus Resource
There are two main classes in boto3 to interact with AWS services:
Client:
The client class provides a low-level interface to AWS services. It requires the explicit setting of the API operations and parameters.
- To connect to the low-level client interface, you must use Boto3’s client(). You then pass in the name of the service you want to connect to, in this case, s3:
import boto3
s3_client = boto3.client('s3')
Both Client and Resource can be used to perform the same operations, but Resource provides a more Pythonic interface to the services and automatically manages AWS service connection pooling and retries, among other things.
So, depending on your requirements, you can choose either the Client or the Resource interface to interact with AWS services in your applications.
When it comes to choosing between Client and Resource in boto3 for interacting with AWS services, it’s important to understand the differences between the two.
The Client class provides a low-level interface to AWS services and requires more programmatic work on the developer’s part. Responses are returned as dictionaries, and the developer must parse them to extract the desired information. Although using the Client class may offer slight performance improvements, it can make the code less readable.
On the other hand, the Resource class provides a higher-level, more Pythonic interface to AWS services. The SDK automatically handles tasks such as connection pooling and retries, making the code easier to comprehend.
It’s also important to note that Client and Resource are generated from different definition files in boto3. While the Client class supports every type of interaction with the target AWS service, the Resource class may not support every operation available in the Client. However, if an operation is only supported by the Client, it can still be accessed directly through the Resource using s3_resource.meta.client.
One example of a Client operation not supported by the Resource is .generate_presigned_url(), which allows you to grant access to an object in your bucket without requiring AWS credentials for a set period of time.
Common Operations
With an understanding of the distinction between clients and resources, we can now commence constructing new S3 components using them.
Creating a Bucket
The name of an Amazon S3 bucket must be unique across all regions of the AWS platform. The bucket can be located in a specific region to minimize latency or to address regulatory requirements.
import boto3
# First, we need to create a boto3 session to access AWS services.
# In this case, we want to access the S3 service.
session = boto3.Session(
aws_access_key_id="YOUR_ACCESS_KEY_ID",
aws_secret_access_key="YOUR_SECRET_ACCESS_KEY",
region_name="YOUR_REGION_NAME"
)
# Next, we create an S3 client object using the session.
s3 = session.client("s3")
# Then, we specify the name of the bucket we want to create.
bucket_name = "your-bucket-name"
# Finally, we use the create_bucket method of the S3 client object to create the bucket.
response = s3.create_bucket(
Bucket=bucket_name,
CreateBucketConfiguration={
"LocationConstraint": "YOUR_REGION_NAME"
}
)
# The response will contain information about the newly created bucket.
print(response)
With an understanding of the distinction between clients and resources, we can now commence constructing new S3 components using them. In this code, we start by creating a boto3 session using boto3.Session class. The session requires your AWS access key ID, secret access key, and the region in which you want to create the S3 bucket.
Next, we create an S3 client object using the session.client(“s3”) method. The S3 client is what we’ll use to interact with the S3 service.
We then specify the name of the bucket we want to create and store it in the bucket_name variable.
Finally, we use the create_bucket method of the S3 client object to create the bucket. The method requires that you specify the name of the bucket (Bucket=bucket_name), and the location constraint of the bucket, which indicates the region in which the bucket should be created.
The create_bucket method returns a response object, which will contain information about the newly created bucket. You can print the response object to see its contents.
Creating an EC2 Instance
The following code examples show how to get started using Amazon Elastic Compute Cloud (Amazon EC2).
import boto3
# First, we need to create a boto3 session to access AWS services.
# In this case, we want to access the EC2 service.
session = boto3.Session(
aws_access_key_id="YOUR_ACCESS_KEY_ID",
aws_secret_access_key="YOUR_SECRET_ACCESS_KEY",
region_name="YOUR_REGION_NAME"
)
# Next, we create an EC2 client object using the session.
ec2 = session.client("ec2")
# Then, we specify the parameters for the instance we want to create, such as:
# - the Amazon Machine Image (AMI) ID of the image to use for the instance
# - the instance type (e.g. t2.micro)
# - the key pair name for the instance
# - the security group for the instance
instance_params = {
"ImageId": "ami-0c55b159cbfafe1f0",
"InstanceType": "t2.micro",
"MinCount": 1,
"MaxCount": 1,
"KeyName": "your-key-pair-name",
"SecurityGroups": [
"your-security-group-name"
]
}
# Finally, we use the run_instances method of the EC2 client object to launch the instance.
response = ec2.run_instances(**instance_params)
# The response will contain information about the newly created instance(s).
print(response)
In this code, we start by creating a boto3 session using boto3.Session class. The session requires your AWS access key ID, secret access key, and the region in which you want to create the EC2 instance.
Next, we create an EC2 client object using the session.client(“ec2”) method. The EC2 client is what we’ll use to interact with the EC2 service.
We then specify the parameters for the instance we want to create in a dictionary named instance_params. The required parameters include the Amazon Machine Image (AMI) ID of the image to use for the instance, the instance type (e.g. t2.micro), the number of instances to launch (MinCount and MaxCount), the key pair name for the instance, and the security group for the instance.
Finally, we use the run_instances method of the EC2 client object to launch the instance. The method requires that you pass in the instance parameters as arguments (**instance_params).
The run_instances method returns a response object, which will contain information about the newly created instance(s). You can print the response object to see its contents.
Resource:
The resource class provides a higher-level interface to AWS services. It provides a more abstract interface to the service, which maps more closely to the underlying service model.
- To connect to the high-level interface, you’ll follow a similar approach, but use the resource():
import boto3
s3_resource = boto3.resource('s3')
Resources offer a more user-friendly output compared to Clients, once you get the hang of it. The documentation states that Resources can be broken down into several elements such as identifiers, attributes, actions, references, sub-resources, and collections.
Identifiers:
Identifiers refer to the properties of a resource that are set when the resource is first created. These properties act as a unique identifier for the resource and are used to distinguish it from other resources.
For example, when creating an Amazon S3 bucket, the bucket name is set as an identifier for the resource. This name acts as the unique identifier for the bucket and is used in all subsequent operations on that bucket, such as uploading or retrieving objects from the bucket.
Attributes:
Attributes refer to the properties of a resource that provide information about that resource. They are loaded the first time they are accessed via the load() method. Attributes are used in conjunction with a resource’s identifier to retrieve information about the specified resource.
For example, when working with an EC2 instance, the instance ID can be used to retrieve information about the Amazon Machine Image (AMI) that the instance is running. This information can be accessed by calling the image_id attribute and passing in the instance ID as an argument.
References:
References refer to related resource instances that have a parent-child relationship. Unlike Attributes, which require a resource’s identifier to access, References are methods that belong to the Python object representing the resource and do not need the identifier to be provided.
For example, when working with Amazon S3, a bucket can have multiple objects stored in it. The relationship between the bucket and its objects can be viewed as a parent-child relationship, where the bucket is the parent and the objects are the children. In this case, the bucket instance would have a reference method to retrieve its related objects, without requiring the bucket’s identifier to be provided.
Actions:
Actions refer to operations that can be performed on resources. These operations allow you to manipulate the resources in some way, such as creating, updating, or deleting a resource. Actions are methods of the Python object representing the resource, and they may automatically handle the passing in of arguments set from identifiers and some attributes.
For example, when working with Amazon EC2, you can perform actions such as starting an instance or terminating an instance. These actions are performed by calling methods on the EC2 instance object, and the necessary arguments, such as the instance ID, can be automatically passed in based on the identifier and attributes of the instance.
Collections:
Collections refer to a group of resources that can be manipulated and iterated over. They provide an interface for working with multiple resources in a more convenient and organized manner.
For example, when working with Amazon EC2, you may want to iterate over all the IP addresses in a Virtual Private Cloud (VPC) or retrieve the number of volumes attached to an instance. Collections provide a way to easily access and manipulate these groups of resources, rather than having to perform operations on each resource individually.
Here is an example of how to create an EC2 resource using a boto3 session in Python:
import boto3
# create a session
session = boto3.Session(
region_name="us-west-2",
aws_access_key_id="ACCESS_KEY",
aws_secret_access_key="SECRET_KEY"
)
# create an EC2 resource
ec2 = session.resource("ec2")
# create an EC2 instance
instance = ec2.create_instances(
ImageId="ami-0c55b159cbfafe1f0",
MinCount=1,
MaxCount=1,
InstanceType="t2.micro"
)
# retrieve the instance ID
instance_id = instance[0].id
# retrieve the instance's image ID
image_id = instance[0].image_id
# start the instance
instance[0].start()
# terminate the instance
instance[0].terminate()
In this example, we create a session using boto3.Session and provide the necessary credentials (access key and secret key) and the desired region. Then, we create an EC2 resource using session.resource(“ec2”).
We then create an EC2 instance using the create_instances method on the EC2 resource. After creating the instance, we retrieve its instance ID and image ID using the id and image_id attributes, respectively.
Finally, we perform actions on the instance, such as starting and terminating the instance, using the start and terminate methods, respectively.
In this example, we can see how boto3 resources make it easier to work with AWS services by providing a convenient interface to access and manipulate resources.
When using the Client, the output provides a large number of details about the instance, but when using a Resource, the output only shows the instance’s ID. In order to obtain more information about the instance, we need to extract the ID and use it to retrieve the information we need.
In conclusion, boto3 is a powerful tool for AWS developers that provides a user-friendly and flexible way to interact with AWS services. Whether building simple scripts or complex applications, boto3 is essential for anyone working with AWS. With its focus on performance and efficiency, AWS has set a new standard in cloud development, and boto3 is the key to unlocking the full potential of AWS services.