What is Jenkins:
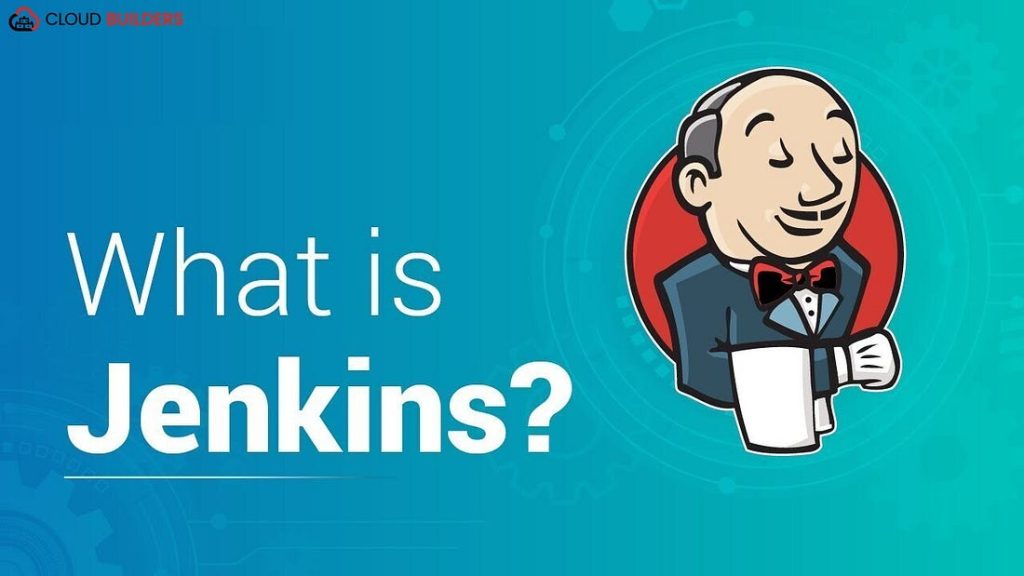
Jenkins is a free and open-source automation server that provides a platform for continuous integration and continuous delivery (CI/CD). It helps developers to automate the process of building, testing and deploying software.
Jenkins is a server-based application, and it requires a server to run. It can run on a variety of operating systems, including Windows, Linux, and macOS.
The primary use case for Jenkins is to automate the build and testing process for software projects. When developers make changes to the source code, Jenkins can automatically compile the code, run unit tests, and package the software into a deployable format. This helps to catch bugs early in the development process and reduces the time it takes to get changes into production.
Jenkins also provides a web interface for creating and managing build jobs. This web interface allows developers to create and configure build jobs, view build results, and manage the build process. The interface also provides tools for monitoring and analyzing build results and performance.
Jenkins integrates with many different source code management (SCM) systems, including Git, Subversion, and Mercurial. This integration allows developers to trigger builds automatically when changes are committed to the source code repository.
Jenkins also supports a large and active community of developers and users. This community has developed hundreds of plugins that extend the functionality of Jenkins. These plugins provide support for a wide range of technologies, including cloud platforms, testing frameworks, and deployment tools.
Jenkins pipelines:
A Jenkins pipeline is a series of steps, defined in a Jenkinsfile, that represent the entire build process for a software project. It is used to automate the continuous integration and continuous delivery (CI/CD) of applications.
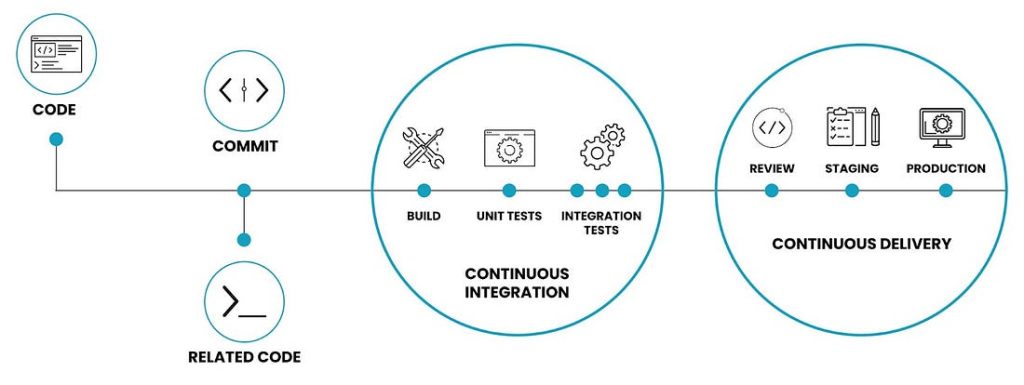
A Jenkins pipeline consists of a series of stages, each of which represents a step in the build process. Each stage consists of one or more tasks, such as building the software, running tests, or deploying the software to a production environment. The pipeline can be visualised as a directed acyclic graph (DAG) with the stages as nodes and the tasks as edges.
The pipeline is defined in a Jenkinsfile, which is a text file that is checked into the source code repository for the project. This allows the pipeline to be versioned along with the source code, and it makes it easy to update and maintain the pipeline over time.
Jenkins pipelines support many different types of tasks, including shell scripts, shell commands, and the execution of scripts written in other programming languages. The pipelines can also be integrated with other tools and services, such as source code management systems, testing frameworks, and deployment tools.
One of the benefits of using a Jenkins pipeline is that it provides a clear and concise view of the entire build process, making it easy to see what has been done and what needs to be done next. Additionally, pipelines provide a high level of control over the build process, allowing developers to define complex build processes and ensure that the correct steps are executed in the correct order.
Types of Jenkins pipeline
There are two main types of Jenkins pipelines:
Declarative Pipelines:
Declarative pipelines were introduced in Jenkins 2.0 and provided a simplified way to define CI/CD pipelines. Declarative pipelines use a declarative syntax, which is easier to read and understand than the traditional Groovy scripts used in scripted pipelines.
The declarative pipeline syntax defines the stages and steps of a pipeline in a straightforward and intuitive way. The pipeline is defined in a Jenkinsfile, which is stored in the source code repository for the project. The pipeline is executed by the Jenkins server, which runs the stages and steps defined in the Jenkinsfile.
Declarative pipelines provide a number of built-in steps, such as build, test, and deploy, which can be used to define the CI/CD process for a project. Additionally, declarative pipelines provide a number of advanced features, such as parallelism, error handling, and conditional execution, which can be used to create complex and flexible build processes.
Declarative pipelines are recommended for most use cases, as they provide a simple and easy-to-use syntax that makes it easy to define and maintain CI/CD pipelines.
Here is an example of a simple declarative pipeline in Jenkins:
pipeline {
agent { label 'nodejs' }
stages {
stage('Build') {
steps {
echo 'Building the application…'
sh 'npm install'
}
}
stage('Test') {
steps {
echo 'Testing the application…'
sh 'npm test'
}
}
stage('Deploy') {
steps {
echo 'Deploying the application…'
sh 'npm run deploy'
}
}
}
}
In this example, the pipeline is defined in a Jenkinsfile and consists of three stages: Build, Test, and Deploy. The pipeline is executed on a Jenkins node with the label ‘NodeJS’.
The Build stage uses a sh step to run the npm install command, which installs the dependencies for the application. The Test stage uses a sh step to run the npm test command, which runs the tests for the application. The Deploy stage uses a sh step to run the npm run deploy command, which deploys the application.
This is a simple example of a declarative pipeline in Jenkins, but it demonstrates the basic syntax and structure of a declarative pipeline.
Scripted Pipelines:
Scripted pipelines, also known as traditional pipelines, use a Groovy script to define the stages and steps of a CI/CD pipeline. The pipeline is defined in a Jenkinsfile, which is stored in the source code repository for the project.
Scripted pipelines offer more flexibility and control than declarative pipelines, as the Groovy script can be used to define complex and custom-build processes. However, they also require a deeper understanding of the Groovy language and can be more difficult to read and maintain than declarative pipelines.
Scripted pipelines provide a number of advanced features, such as loops, variables, and functions, which can be used to create complex and flexible build processes. Additionally, scripted pipelines can be integrated with other tools and services, such as source code management systems, testing frameworks, and deployment tools.
Scripted pipelines are recommended for more complex and custom build processes, as they provide more flexibility and control than declarative pipelines. However, they require a deeper understanding of the Groovy language and can be more difficult to read and maintain.
Here is an example of a simple scripted pipeline in Jenkins:
node {
stage('Build') {
echo 'Building the application…'
sh 'npm install'
}
stage('Test') {
echo 'Testing the application…'
sh 'npm test'
}
stage('Deploy') {
echo 'Deploying the application…'
sh 'npm run deploy'
}
}
In this example, the pipeline is defined in a Jenkinsfile and consists of three stages: Build, Test, and Deploy. The node block specifies the Jenkins node that will be used to execute the pipeline.
The Build stage uses a sh step to run the npm install command, which installs the dependencies for the application. The Test stage uses a sh step to run the npm test command, which runs the tests for the application. The Deploy stage uses a sh step to run the npm run deploy command, which deploys the application.
This is a simple example of a scripted pipeline in Jenkins, but it demonstrates the basic syntax and structure of a scripted pipeline. Note that the pipeline can be extended and customized using the full power of the Groovy language, making it possible to define complex and custom-build processes in Jenkins.
In conclusion, Jenkins is a powerful and versatile tool that helps developers to automate the software development process. With its support for multiple operating systems, SCMs, and plugins, it is a popular choice for CI/CD automation.