A Beginner’s Guide
Main Features of Terraform
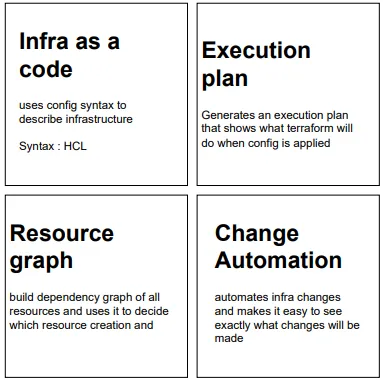
How It Works
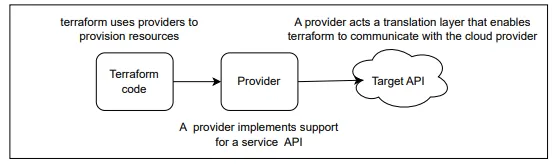
The Core Workflow
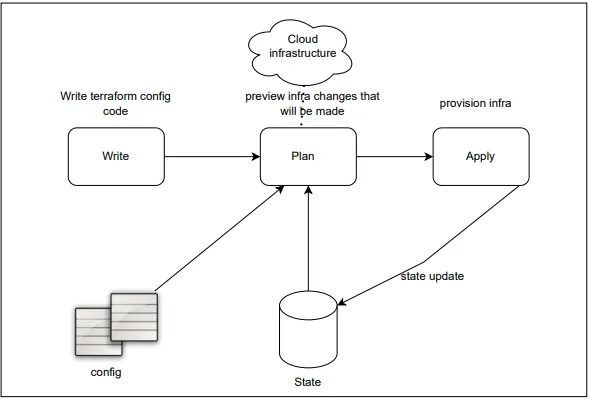
The terraform basic CLI.
Running terraform You usually run terraform using the CLI in a directory that contains. tf files.
[Terraform init]
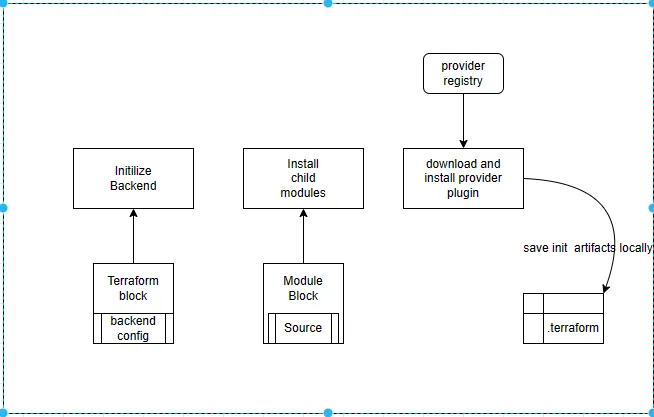
[Terraform Plan]
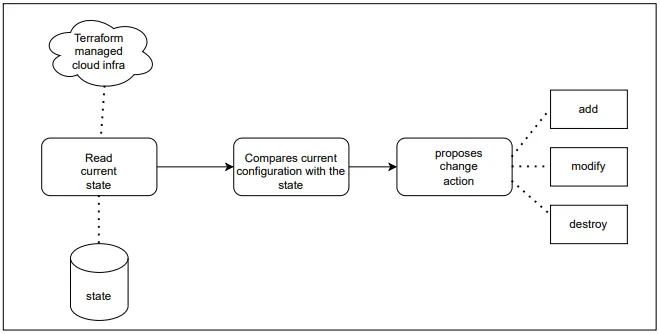
[Terraform Apply]
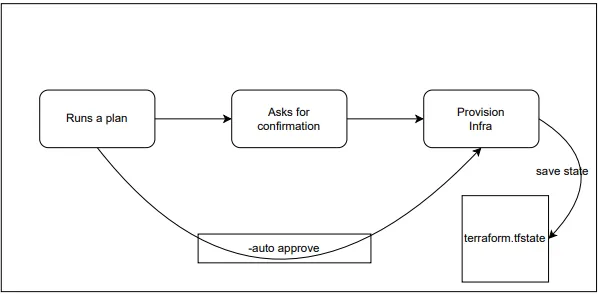
What is IAC
Infrastructure as Code it is the process of managing infrastructure in a file or files rather than manually configuring resources in a user interface. A resource in this instance is any piece of infrastructure in a given environment, such as a virtual machine, security group, network interface, etc.
Terraform is a tool for building, changing, and versioning infrastructure safely and efficiently. Terraform can manage existing and popular service providers as well as custom in-house solutions.
Configuration files describe to Terraform the components needed to run a single application or your entire datacenter. Terraform generates an execution plan describing what it will do to reach the desired state, and then executes it to build the described infrastructure. As the configuration changes, Terraform is able to determine what changed and create incremental execution plans which can be applied.
The infrastructure Terraform can manage includes low-level components such as compute instances, storage, and networking, as well as high-level components such as DNS entries, SaaS features, etc.
Manage Infrastructure
Variable type
Strings, Numbers, Boolean, List, or Maps.
- Note: Number or integers don’t need double quotes, but Terraform automatically converts number and bool values to strings when needed. For example 5 and “5” both are correct.
Variable List
List is the same as an array. We can store multiple values Remember the first value is the 0 position. For example to access the 0 position is var.mylist[0]
Variable Map
Is a Key:Value pair. We use the key to access to the value
Important: For example, if we need to access the value of Key1 (Value1) we can using the next example var.mymap[“Key1”]
- Note: Remember, we use [ ] for list, and we use { } for maps
TFVARS FILES
Passing variables inside a file, this is possible create a file called terraform.tfvars this file can be in a yaml or json notation, and is very simple, and also we can add maps
- Note: The terraform.tfvars file is used to define variables and the .tf file declares that the variable exists.
MULTIPLE VALUE FILES
We can create a specified *.tvars file and load for example with terraform plan, this is very useful to settings variables for different environments.
[terraform plan -var-file=prod.tfvars]
VERSIONING
The required_version setting can be used to constrain which versions of the Terraform CLI can be used with your configuration. If the running version of Terraform doesn’t match the constraints specified, Terraform will produce an error and exit without taking any further actions.
PROVIDERS
A provider is responsible for understanding API interactions and exposing resources. If an API is available, you can create a provider. A provider uses a plugin. In order to make a provider available on Terraform, we need to make a terraform init, this commands download any plugins we need for our providers. If for example we need to copy the plugin directory manually, we can do it, moving the files to .terraform.d/plugins
- Note: Using terraform providers command we can view the specified version constraints for all providers used in the current configuration
TERRAFORM INIT
The terraform init command is used to initialize a working directory containing Terraform configuration files. It is safe to run this command multiple times, this command will never delete your existing configuration or state. During init, the root configuration directory is consulted for backend configuration and the chosen backend is initialized using the given configuration settings.
TERRAFORM VALIDATE
The terraform validate command validates the configuration files in a directory, referring only to the configuration and not accessing any remote services such as remote state, provider APIs, etc.
- Note: Validation requires an initialized working directory with any referenced plugins and modules installed.
TERRAFORM PLAN
The terraform plan command is used to create an execution plan. Terraform performs a refresh, unless explicitly disabled, and then determines what actions are necessary to achieve the desired state specified in the configuration files.
TERRAFORM APPLY
The terraform apply command is used to apply the changes required to reach the desired state of the configuration, or the predetermined set of actions generated by a terraform plan execution plan.
TERRAFORM DESTROY
The terraform destroy command is used to destroy the Terraform-managed infrastructure.
LEARN MORE SUBCOMMANDS
FMT
The terraform fmt command is used to rewrite Terraform configuration files to a canonical format and style. The canonical format may change in minor ways between Terraform versions, so after upgrading Terraform it is recommended to proactively run fmt.
TAINT
The terraform taint command manually marks a Terraform-managed resource as tainted, forcing it to be destroyed and recreated on the next apply. Taint force the recreation. This command will not modify infrastructure, but does modify the state file in order to mark a resource as tainted. Once a resource is marked as tainted, the next plan will show that the resource will be destroyed and recreated and the next apply will implement this change.
Forcing the recreation of a resource is useful when you want a certain side effect of recreation that is not visible in the attributes of a resource. For example: re-running provisioners will cause the node to be different or rebooting the machine from a base image will cause new startup scripts to run.
UNTAINT
The terraform untaint command manually unmarks a Terraform-managed resource as tainted, restoring it as the primary instance in the state.
- Note: This command will not modify infrastructure but does modify the state file in order to unmark a resource as tainted.
IMPORT
The terraform import command is used to import existing resources into Terraform.
Import will find the existing resource from ID and import it into your Terraform state at the given ADDRESS.
ADDRESS must be a valid resource address. Because any resource address is valid, the import command can import resources into modules as well directly into the root of your state.
ID is dependent on the resource type being imported. For example, for AWS instances it is the instance ID (i-abcd1234) but for AWS Route53 zones it is the zone ID (Z12ABC4UGMOZ2N).
SHOW
The terraform show command is used to provide human-readable output from a state or plan file. This can be used to inspect a plan to ensure that the planned operations are expected, or to inspect the current state as Terraform sees it.
Usage: terraform show [options] [path]
You may use show with a path to either a Terraform state file or plan file. If no path is specified, the current state will be shown.
WORKSPACE INTRODUCTION
The terraform workspace command is used to manage workspaces. It is useful to split and separate the state files.
This command is a container for further subcommands such as list, select, new, delete and show.
Usage: terraform workspace <subcommand> [options] [args]
If we never create a workspace, we use the default workspace.
- Note: For local state, Terraform stores the workspace states in a directory called terraform.tfstate.d. This directory should be treated similarly to local-only terraform.tfstate
- Note: Terraform Cloud and Terraform CLI both have features called “workspaces,” but they’re slightly different. CLI workspaces are alternate state files in the same working directory; they’re a convenience feature for using one configuration to manage multiple similar groups of resources.
Manage state.
LOCAL STATE
The local backend stores state on the local filesystem, locks that state using system APIs, and performs operations locally.
terraform {
backend “local” {
path = “relative/path/to/terraform.tfstate”
}
}
REMOTE BACKENDS
Stores the state as a given key in a given bucket on Amazon S3. This backend also supports state locking and consistency checking via Dynamo DB, which can be enabled by setting the DynamoDB Table field to an existing DynamoDB table name. A single DynamoDB table can be used to lock multiple remote state files. Terraform generates key names that include the values of the bucket and key variables.
Example:
terraform {
backend “s3” {
bucket = “mybucket”
key = “state/terraform.tfstate”
region = “us-east-1”
}
}
- Note: This assumes we have a bucket created called mybucket. The Terraform state is written to the key state/terraform.tfstate.
- Important: If we have a local state, and after that we change to the S3 backend, when we execute terraform init the system ask us if we want to copy existing state to the new backend.
REVERT TO LOCAL BACKEND
If we want to change from S3 backend to Local backend, only we need to do terraform destroy after that delete backend.tf file, and run terraform init
BACKEND LIMITATIONS & SECURITY
When we use Terraform is only allowed one backend.
The state cannot store secrets, for that reason we need to encrypt at rest.
TYPES OF BACKEND
Standard: state management, storage and locking
Enhanced: Only on Terraform Cloud, standard + can run operations remotely
- Note: Backend that support state lockings: Azurerm, Consul, S3